On Linux systems (Centos, Fedora, Ubuntu, Mint, Puppy), a running application is identified by one or more processes. Example when running a document with LibreOffice Writer, the process thats related is oosplash and soffice.bin as can be seen below.
tboxmy 5262 0.3 0.0 299672 3272 ? Sl 10:17 0:00 /usr/lib64/libreoffice/program/oosplash --writer
tboxmy 5277 3.1 1.1 1273804 89564 ? Sl 10:17 0:01 /usr/lib64/libreoffice/program/soffice.bin --writer --splash-pipe=5
Shown in bold, the unique process identification (id) are
5262 and
5277 for the two processes. Upon using the command ps xl, it can be seen that process
5277 is spawned by process
5262. Making
5262 a parent to
5277.
What is a defunct process?
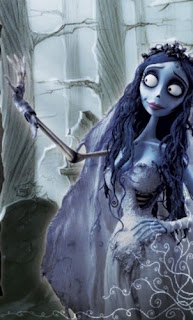
A "
defunct" processes is referred as a dead process that still hogs memory resources. Its not much a problem having a defunct process, but when the system starts to have more defunct processes, the system can slow down and affect applications.
This defunct or dead process that still lingers on the system is also known as
zombie process. Most appropriate, like in a horror flick! You can't kill a zombie that is already dead, so what can you do? Watch the next zombie show and try figuring that out. Luckily, on Linux systems, a zombie doesn't go around attacking or destroying resources. It only lingers on, hogging on to resources once given to it when it was a live process, at one time.
What brings about this zombie? On Linux its mostly due to bad programming that lead to errors and bugs that are not handled by the application itself.
Dealing with defunct process
Don't attempt to bring back to live a zombie process. Seek out the zombie and terminate from the system. Here are commands to handle an example zombie processes (defunct) with process id
5277.
Where are the zombie processes?
$ ps ef |grep defunct
5277 ? Z 0:01 [example-app] <defunct>
Notice the word "defunct" and status as Z (for zombie) for the process
5277. That, is our zombie. Did you find any zombie's in your system?
The command kill is used to terminate any process and free system resources such a memory (RAM). The problem with zombie processes is that, they are dead, and can't be killed (not most of the time). What is done however, is to identify the parent process (the one responsible for creating the zombie) and terminate that process.
Identify the parent process id (5262) and terminate it.
$ ps -o ppid 5277
PPID
5262
$ kill -9 5262
$ ps ef |grep defunct
That is the end of the zombie. In some cases, a zombie might be holding on to a resource and it will take sometime for it to detach (give it a bit of a wait).
There are other methods to look for zombies. E.g. the ps command provides a method of displaying the parent hierarchy as follows;
$ ps xlf |grep defunct
Or in the command
top, look at the second line for number of zombie processes.
What if the zombie keeps appearing on a daily basis for the same process?
This is a problem, but sometimes its not apparent as zombie's live among the running processes without bothering anyone. Let me know if you have other than the suggestions I have list below.
- See if there is a newer version of the application or an alternative (such as different architecture, from a different source) and install.
- It is possible that the application itself is not the problem. Identify any other related applications or module that is causing this zombie. Then update or find an alternative application to use.
- Report to the application project team for a solution.